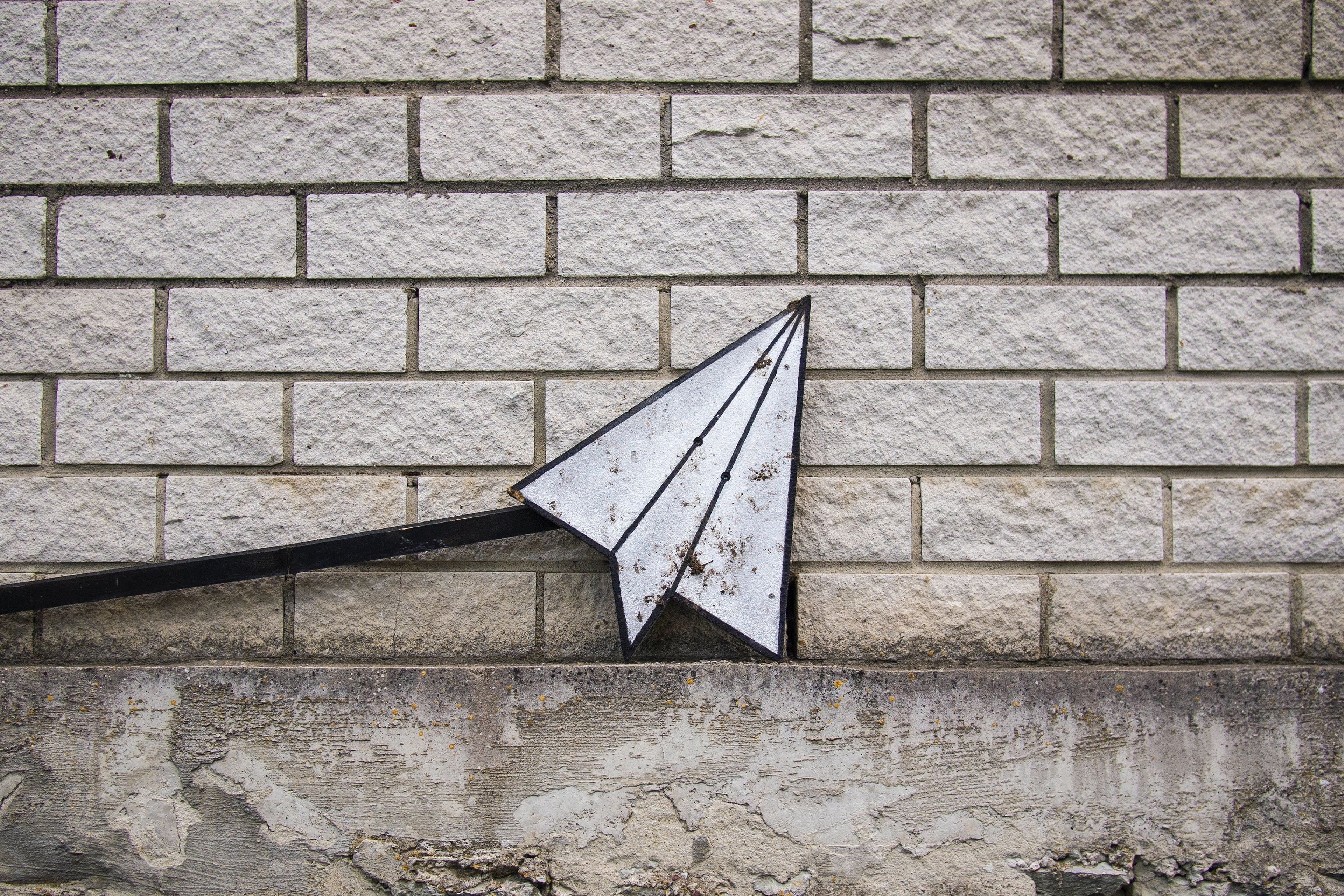
Published: Apr 22, 2022
Last Updated: Mar 9, 2024
How to send emails from Nextjs without the Backend using EmailJs
While working on a client-side project, I encountered the challenge of implementing a contact form without relying on server-side code. This is where EmailJs comes to the rescue. In this article, I'll walk you through the process of sending emails directly from your Next.js app using EmailJs.
Understanding EmailJs and Its Benefits
EmailJs is a powerful platform that allows you to send emails directly from your client-side JavaScript code, eliminating the need for server-side processing. With features like static and dynamic attachments, as well as support for dynamic parameters, EmailJs offers a user-friendly solution. Its comprehensive documentation and modern technology support make it an excellent choice for seamlessly integrating email functionality into your projects.
Covered Topics
Setting Up an EmailJs Account
Retrieving Environment from Emailjs dashboard
Setting Up Environment Variables in Next.js
Integrate EmailJs: Building the Contact Page and Enhancing Styles.
How to Set Up Your EmailJs Account
Create an EmailJS Account: Visit the EmailJS website and create an account. You'll need to provide your email address and set up a password. Login to EmailJS Dashboard: After creating your EmailJS account, log in to the EmailJS dashboard using your credentials.
Create a Service: In the EmailJS dashboard, navigate to the "Services" section and click on "Create new service." Follow the prompts to set up the service. You might need to connect your email account by providing relevant details like SMTP settings.
Add Email Templates: After creating a service, you can add email templates. These templates consist of predefined messages you can use in your code. They can include placeholders for dynamic data.
Create a Template: In the EmailJS dashboard, go to the "Templates" section and click on "Create new template." Provide a name, subject, and HTML/CSS content for the email body.
Test the Template: You can preview and test your email template within the EmailJS dashboard. You can even send a test email to your personal address to see how it will appear when received.
Retrieving Environment Variables
To use the correct values for the SERVICE_ID
, TEMPLATE_ID
, and PUBLIC_KEY
environment variables in your Next.js application, you need to access these values within your EmailJS account dashboard. Here's how you can find these values:
SERVICE_ID:
Log in to your EmailJS account dashboard.
Go to the "Email Services" section.
Click on the service that you've set up or want to use.
You will find the "Service ID" listed on this page.
TEMPLATE_ID:
After logging in, navigate to the "Email Templates" section in the EmailJS dashboard.
If you have existing templates, you'll see a list of them. Click on the template you want to use.
On the template details page, you should find the "Template ID."
PUBLIC_KEY:
In your EmailJS account dashboard, look for the "Account" section or something similar.
You should be able to find your "Public API Key" there.
Once you have retrieved these values, you can place them in your .env.local
file in your Next.js project. Remember that these values are sensitive, so keep your .env.local
file secure and never share it publicly or commit it to version control.
Setting Up Environment Variables in Next.js
To ensure the availability of environment variables on the client side, it's crucial to use the prefix NEXT_PUBLIC_
. Variables without this prefix are limited to the Node.js environment and cannot be accessed by the browser. This differentiation arises from the distinct environments. Our goal is to enable the use of these variables for tasks such as sending emails directly from the client side.
Create a .env.local
file: Within your project's root directory, insert the following environment variables, ensuring to replace the placeholders with your specific values.
NEXT_PUBLIC_EMAILJS_SERVICE_ID=YOUR_SERVICE_ID
NEXT_PUBLIC_EMAILJS_TEMPLATE_ID=YOUR_TEMPLATE_ID
NEXT_PUBLIC_EMAILJS_USER_ID=YOUR_PUBLIC_KEY
To retrieve these variables, you can utilize the syntax: process.env.VARIABLE_NAME
.
For example:
process.env.NEXT_PUBLIC_EMAILJS_SERVICE_ID
.
Integrate EmailJs: Building the Contact Page and Enhancing Styles.
To proceed, create a new Next.js app or use your existing project. For a new Next.js application, you can follow a tutorial on the Next.js website. Alternatively, I'll be creating a Next.js application on CodeSandbox.
Install the EmailJs package:
npm install @emailjs/browser
# or
yarn add @emailjs/browser
To enhance the visual appeal of our Contact page, we'll create a dedicated stylesheet. Follow these steps to add custom styles to the form:
Create a Stylesheet:
Inside the
src/styles
folder, create a new file namedContact.module.css
.
Copy and Paste the Code:
Copy the CSS code provided below and paste it into the newly created
Contact.module.css
file.
.main {
display: flex;
flex-direction: column;
justify-content: space-evenly;
align-items: center;
padding-inline: 6rem;
padding-block: 6rem;
min-block-size: 100vh;
}
.form {
max-inline-size: 600px;
inline-size: 100%;
}
.label {
display: flex;
margin-block-end: 10px;
}
.formGroup {
margin-block-end: 20px;
}
.formGroup label {
display: block;
font-weight: bold;
}
.formGroup input,
.formGroup textarea {
inline-size: 100%;
padding-inline: 10px;
padding-block: 10px;
border-style: solid;
border-color: #ccc;
border-width: 1px;
border-radius: 4px;
}
.formGroup textarea {
resize: vertical;
}
.button[type="submit"] {
background-color: #007bff;
color: white;
border: none;
padding-block: 10px;
padding-inline: 20px;
border-radius: 4px;
cursor: pointer;
inline-size: 100%;
}
.button[type="submit"]:hover[type="submit"]:hover {
background-color: #0056b3;
}
Navigate to the /pages
folder within your Next.js project. Inside it, generate a new file named contact.tsx
and paste the following code snippet into the file:
import React, { useRef } from "react";
import emailjs from "@emailjs/browser";
import Head from "next/head";
import { Inter } from "next/font/google";
import styles from "@/styles/Contact.module.css";
const inter = Inter({ subsets: ["latin"] });
const ContactUs = () => {
const form = useRef(null);
const sendEmail = (event: React.FormEvent<HTMLFormElement>) => {
event.preventDefault();
if (
process.env.NEXT_PUBLIC_EMAILJS_SERVICE_ID &&
process.env.NEXT_PUBLIC_EMAILJS_TEMPLATE_ID &&
process.env.NEXT_PUBLIC_EMAILJS_USER_ID &&
form.current
) {
emailjs
.sendForm(
process.env.NEXT_PUBLIC_EMAILJS_SERVICE_ID,
process.env.NEXT_PUBLIC_EMAILJS_TEMPLATE_ID,
form.current,
process.env.NEXT_PUBLIC_EMAILJS_USER_ID
)
.then(
(result) => {
alert(result.text);
},
(error) => {
alert(error.text);
}
);
}
};
return (
<>
<Head>
<title>Contact Us</title>
<link rel="icon" href="/favicon.ico" />
<meta name="description" content="Contact us to get in touch with us" />
<meta name="keywords" content="contact, email, message" />
<meta name="author" content="ali" />
</Head>
<div className={`${styles.main} ${inter.className}`}>
<h1>Contact Us</h1>
<form ref={form} onSubmit={sendEmail} className={styles.form}>
<div className={styles.formGroup}>
<label className={styles.label} htmlFor="user_name">
Name
</label>
<input type="text" id="user_name" name="user_name" required />
</div>
<div className={styles.formGroup}>
<label className={styles.label} htmlFor="email">
Email
</label>
<input type="email" id="email" name="email" required />
</div>
<div className={styles.formGroup}>
<label className={styles.label} htmlFor="message">
Message
</label>
<textarea rows={8} id="message" name="message" required />
</div>
<button className={styles.button} type="submit">
Send
</button>
</form>
</div>
</>
);
};
export default ContactUs;
In this code snippet, we import the necessary modules, including the emailjs
library, which facilitates sending emails. The sendEmail
function is responsible for handling the form submission and utilizing the EmailJS SDK to send the form data. Ensure you have set up the required environment variables for the EmailJS service, template, and user IDs in your project.
Your contact.tsx
page is now created and can be accessed at the URL path "/contact".
Conclusion
We have successfully created a secure and efficient method of sending emails from your Next.js application by utilizing the power of EmailJS and environment variables. This setup promotes good development practices, improves security, and guarantees smooth operation across different environments.
Useful Links
GitHub Repository for this Project - Access the GitHub repository for the project created in this tutorial.